Overview
Modulo is for NUS students who prefer to use a desktop app for managing their school work on a modular level. It helps you to break down your modules into deadlines and track their progress.
Currently Modulo is made for NUS students taking modules this Academic Year AY2019/2020 Semester 2! More importantly, Modulo is optimized for those who prefer to work with a Command Line Interface (CLI) while still having the benefits of a Graphical User Interface (GUI). If you can type fast, Modulo can get your module management tasks done faster than traditional GUI apps.
Summary of contributions
-
Major enhancement: Implemented Deletion function to delete modules, events and deadlines
-
What it does: allows the user to delete modules, events or deadlines
-
Justification: This feature is important as it is an essential function should the user want to delete Modules, Events or Deadlines from Modulo.
-
Highlights: This feature also allows for deletion of multiple Modules and Events at a time. For example while on the Module view,
delete CS
will delete all modules containingCS
. This provides an added convenience for the user should the user want to delete some events / modules containing certain keywords. Modulo will also return the appropriate feedback should the user enter invalid inputs or use the command inappropriately.
-
-
Minor enhancement: Initial logic implementation to switch between left panel views.
-
What it does: Refreshes the list view every time the user switches between module and event views.
-
Justification: This feature was important as our team wanted to ensure that users were able to switch between list views in Modulo.
-
-
Minor enhancement: Implemented FindCommand
-
What it does: allows the user to find modules or events using certain keywords.
-
Justification: This feature is important as it is an essential function should the user want to find modules or events by certain keywords.
-
Highlights: This FindCommand allows additional filtering (not only once). For example if the user wants to search for
tutorial 1
from the moduleCS2103
, the user can first enterfind CS2103
and thenfind tutorial 1
. Modulo will also return the appropriate feedback should the user enter invalid inputs or use the command inappropriately.
-
-
Minor enhancement: Implemented ListCommand
-
What it does: allows the user to list modules or events on the left panel of Modulo.
-
Justification: This feature is important as it allows the user to switch between the module and event view.
-
-
Minor enhancement: Created Module and Event Cards to display the details of events and modules
-
What it does: provides details of modules and events.
-
Justification: This feature is important as it allows the user to see details of modules and events in the list
-
Credit: Modified the Module and Event Cards with help from the original implementation of PersonCard from AddressBook3.
-
-
Code contributed: [tP Code Dashboard]
-
Functional Code contributed:
-
Model: [Model Manager]
-
getFilteredDisplayableList()
-
-
View: [Module List Card][Event List Card]
-
UI: [Module Card][Event Card]
-
Logic: [Delete Command][Find Command][List Command][View Command][Delete Command Parser][List Command Parser][View Command Parser][View Command Parser]
-
-
Test Code Contributed: [Delete Command Test][Add Event Command Test][Add Deadline Command Test][Delete Command Parser Test][Add Deadline Command Parser Test][Done Command Parser Test][Name Contains Keyword Predicate test][List Command Parser Test][Export Command Parser Test][List Command Test][Find Command Parser Test][View Command Parser Test]
Contributions to the User Guide
_Given below are sections I contributed to the User Guide. |
Listing events or modules on the left: list
Note: Given that this command changes the list being listed on the left, it is largely GUI-dependent.
Shows a list of all events or modules in the left panel of Modulo.
This helps you to toggle between the two lists.
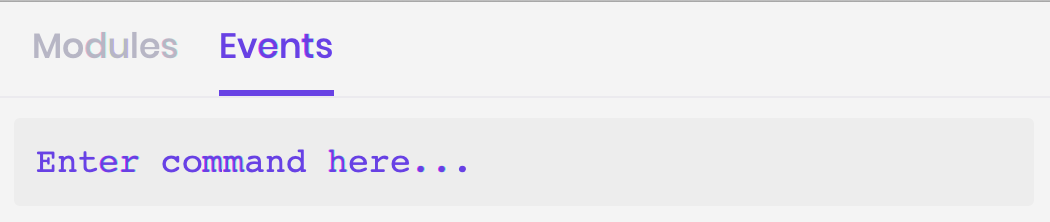
You can always check which list you’re viewing by looking at the menu bar above:
Format: list events
OR list modules
Shorthands:
-
list event
/list e
Same behaviour aslist events
- lists all the events on the left display panel. -
list module
/list m
Same behaviour aslist modules
- lists all the modules on the left display panel.
Finding events or modules using keywords: find
Note: Given that this command searches through the list being listed on the left, it is largely GUI-dependent.
Allows the user to search through the current list for items which match any of the given keywords.
Format: find SEARCH_TERM
Things to note:
-
The search is case insensitive. e.g
find tutorial
will produce all events that match Tutorial. -
Names containing part of the searched words will be matched e.g.
find tut
will match Tutorial 4. -
Modules will be searched according to their Module Code, Name as well as Academic Year.
-
Events will be searched according to their Module Code and Name.
-
After finding, to get back the whole list again, users can simply enter
list events
orlist modules
.
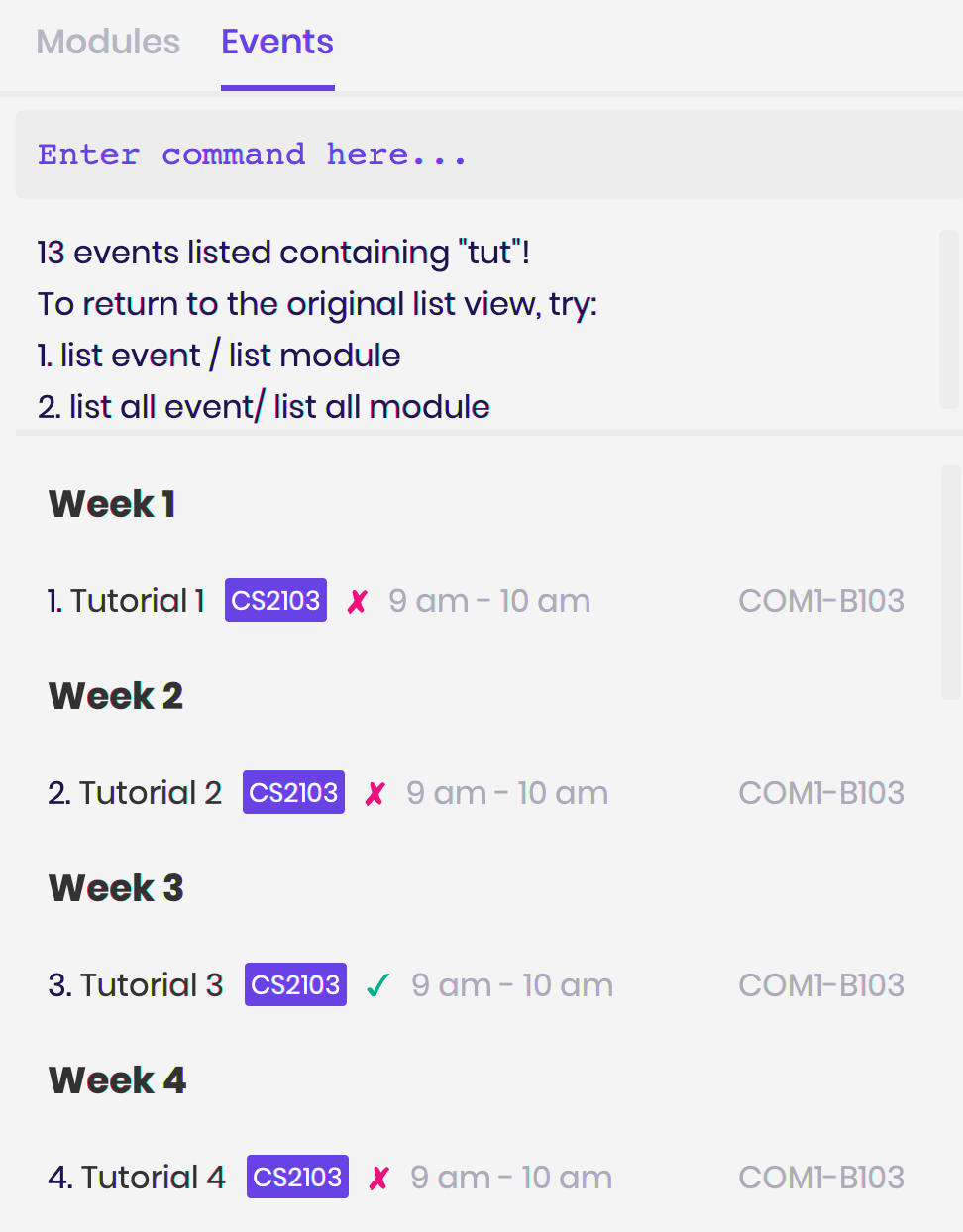
Pro-tip
The find
command allows the user to continually filter their list of searches.
For example, while viewing the list of events, entering find cs2103
and then find tutorial
right after will allow the user to view CS2103 tutorials.
Deleting a module/event/deadline: delete
Note: Given that this command deletes based on the list being listed, it is largely GUI-dependent.
Deletes a module, event, or deadline by its index, a search term, or delete all.
Deleting Modules and Events
Format: delete INDEX
OR delete SEARCH_TERM
OR delete all
Things to note:
-
Deleting a module will delete all its associated events.
-
Deleting an event with delete all its associated deadlines.
-
You can delete a module or an event based on its displayed index on the left panel or using a search term. You can also delete all modules or events.
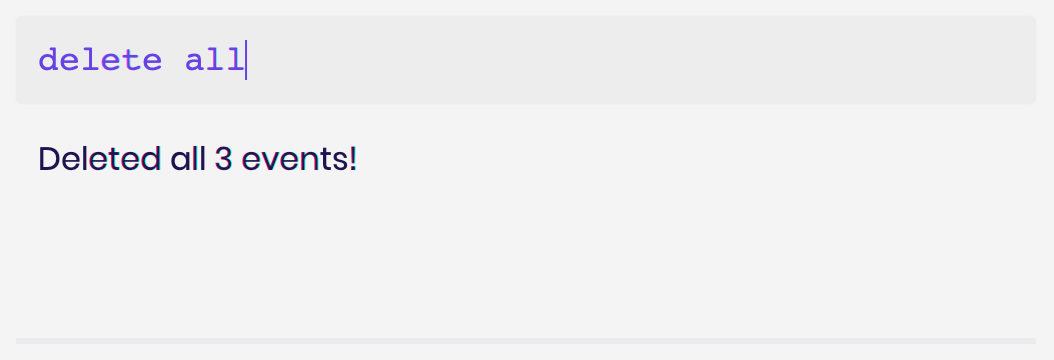
Deleting Deadlines
Note: You NEED to have the event you want focused to do this. We will work on removing the need for this in future versions.
Format: delete d/INDEX
OR delete d/all
Things to note:
-
You can delete an event’s deadline using the deadline’s index.
-
You can also delete all deadlines of that event.
-
This event needs to be currently viewed on the right panel.
Contributions to the Developer Guide
_Given below are sections I contributed to the Developer Guide. |
Architecture
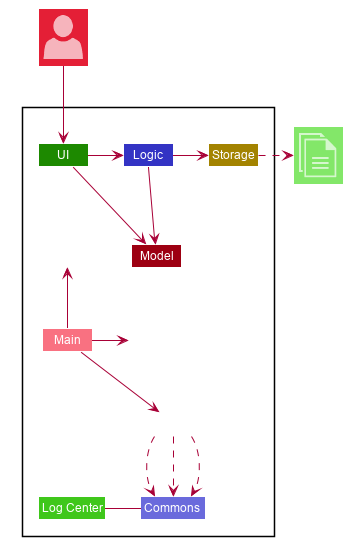
The Architecture Diagram given above explains the high-level design of the App. Given below is a quick overview of each component.
The .puml files used to create diagrams in this document can be found in the diagrams folder.
Refer to the Using PlantUML guide to learn how to create and edit diagrams.
|
-
At app launch: Initializes the components in the correct sequence, and connects them up with each other.
-
At shut down: Shuts down the components and invokes cleanup method where necessary.
Commons
represents a collection of classes used by multiple other components.
The following class plays an important role at the architecture level:
-
LogsCenter
: Used by many classes to write log messages to the App’s log file.
The rest of the App consists of four components.
Each of the four components
-
Defines its API in an
interface
with the same name as the Component. -
Exposes its functionality using a
{Component Name}Manager
class.
For example, the Logic
component (see the class diagram given below) defines it’s API in the Logic.java
interface and exposes its functionality using the LogicManager.java
class.
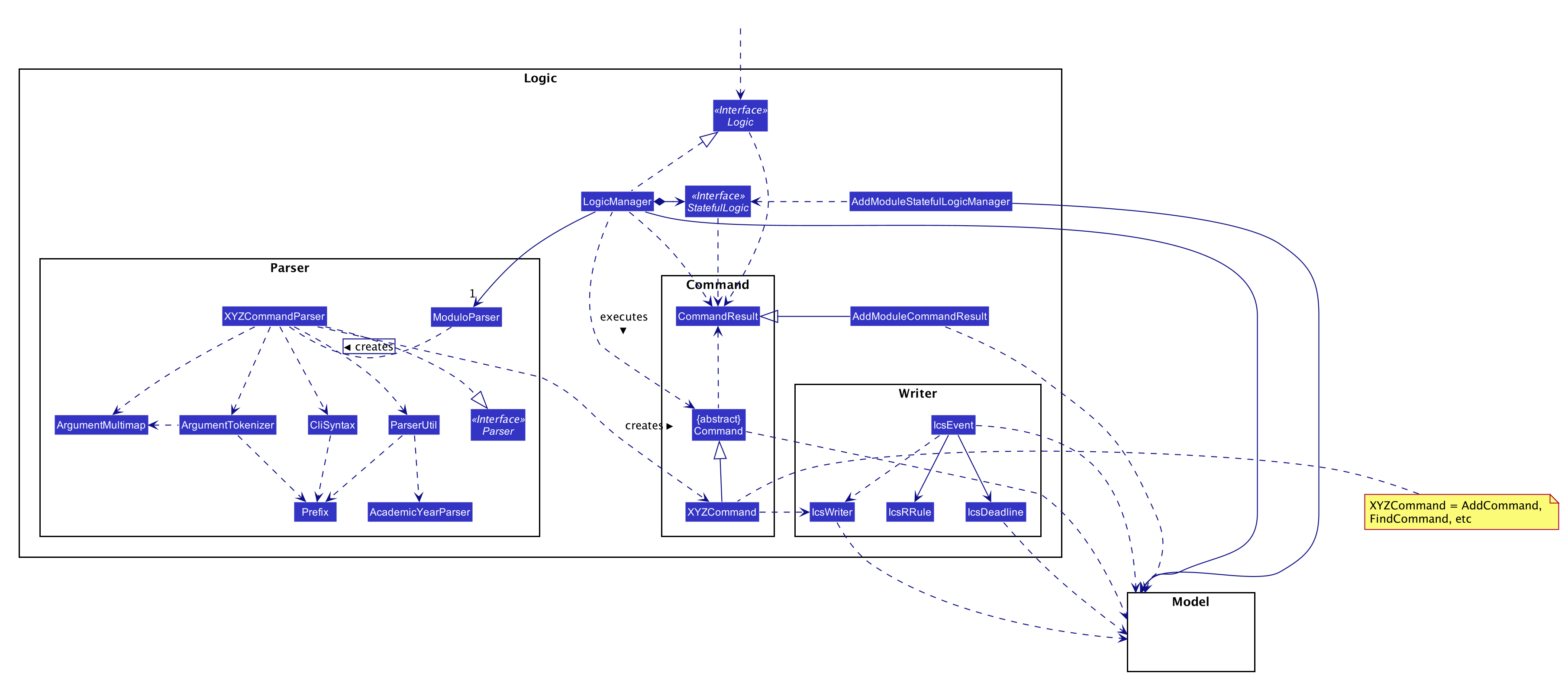
How the architecture components interact with each other
The Sequence Diagram below shows how the components interact with each other for the scenario where the user issues the command delete 1
.
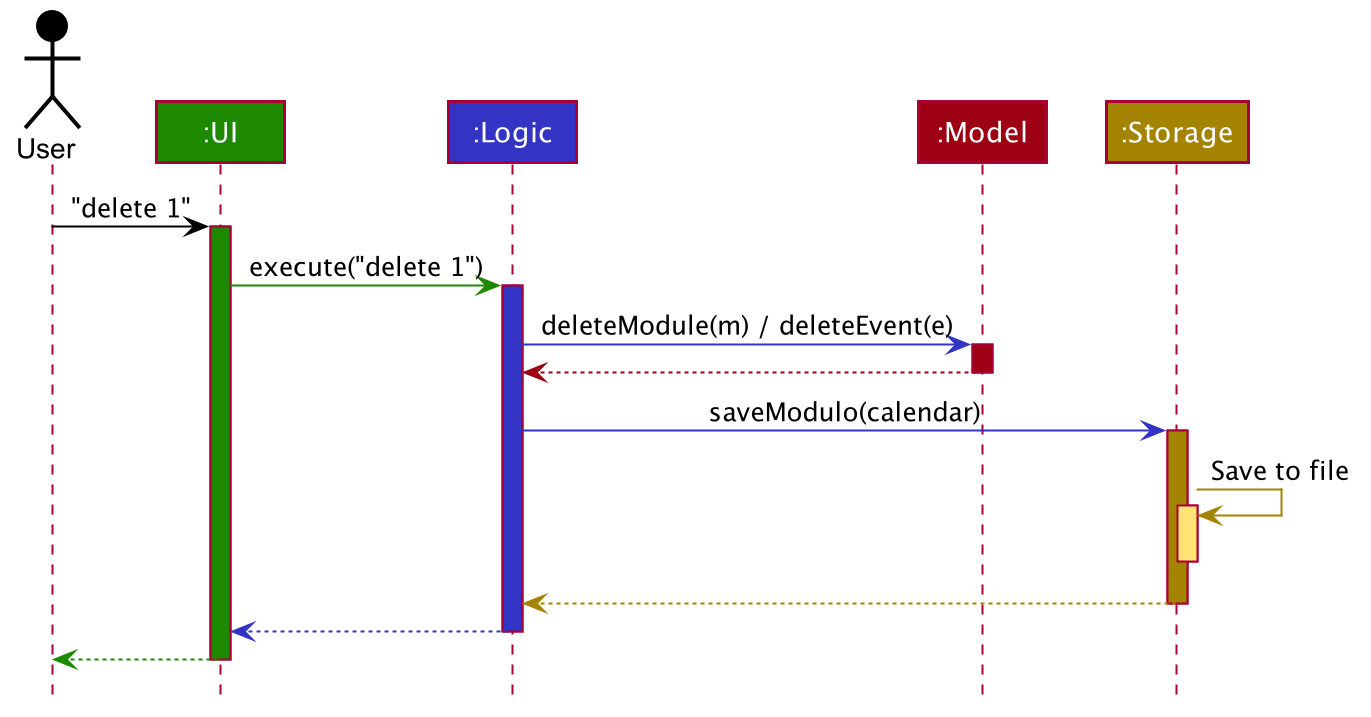
delete 1
commandLogic component
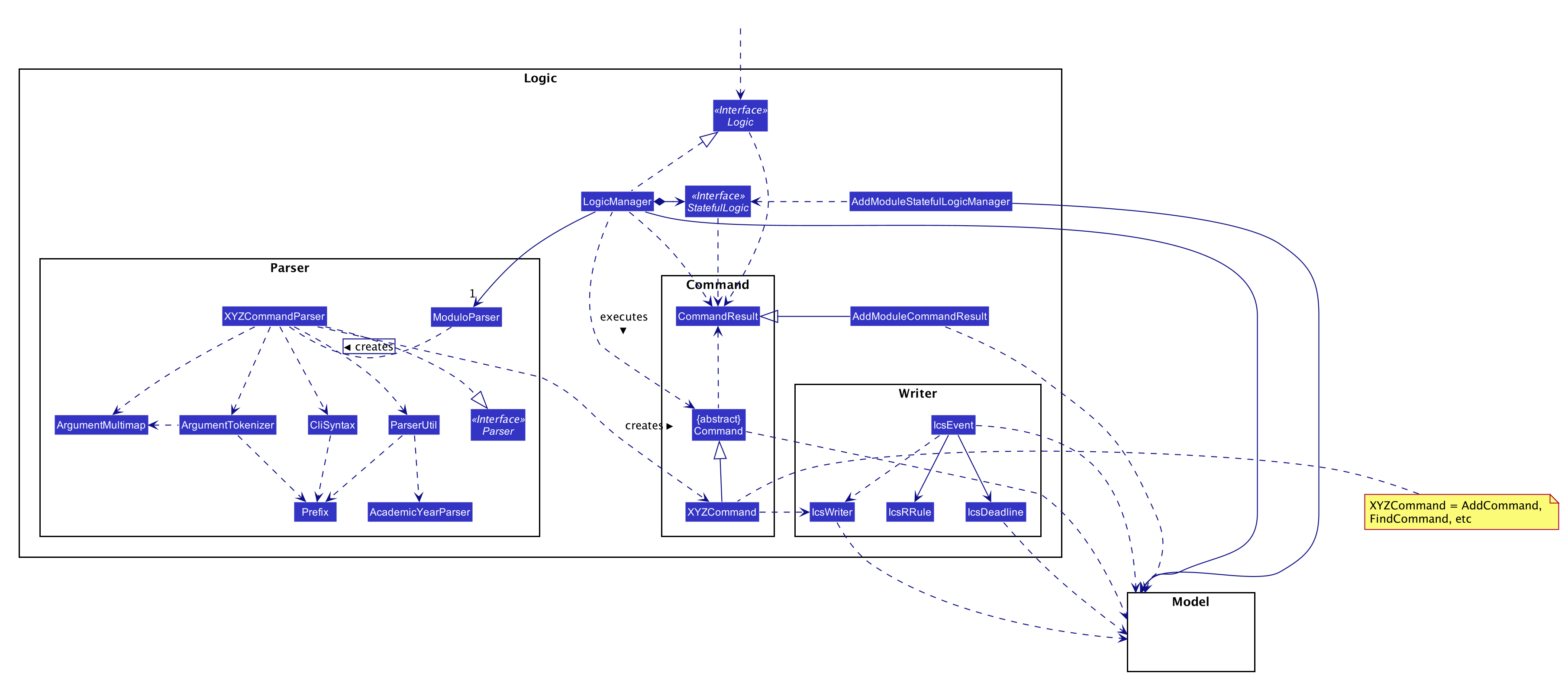
API :
Logic.java
-
Logic
uses theModuloParser
class to parse the user command. -
This results in a
Command
object which is executed by theLogicManager
. -
The command execution can affect the
Model
(e.g. adding an event). -
The result of the command execution is encapsulated as a
CommandResult
object which is passed back to theUi
. -
In addition, the
CommandResult
object can also instruct theUi
to perform certain actions, such as displaying help to the user.
StatefulLogic Interface:
StatefulLogic
is implemented by classes that only manages logic while a state exists.
This state would be loaded from special CommandResult
subclasses, and allows this StatefulLogicManager
to take priority over the default LogicManager
.
The exact implementation of state is up to the developer.
Currently, there is only one class that implements it: AddModuleStatefulLogicManager
.
Its state is loaded from a special AddModuleCommandResult
created by the AddModuleCommand
, i.e. when the user uses the
module m/MODULE_CODE
command.
The state is created using a list of the event types (tutorial, lecture, lab etc.) that the added module has.
Subsequently, while the list is not empty, the AddModuleStatefulLogicManager
handles all inputs, thus allowing Modulo to add the relevant events.
More details on the implementation can be found here.
Writer Component:
The Writer component is activated when the ExportCommand
is executed.
It then calls the writeIcsFile()
function from the IcsWriter
class which retrieves the current list of events from the Model
component which, along with its deadlines, are converted into IcsEvent
and IcsDeadline
objects, which are then written into the ICS file.
Sample Interactions
Given below is the Sequence Diagram for interactions within the Logic
component for the execute("delete 1")
API call.
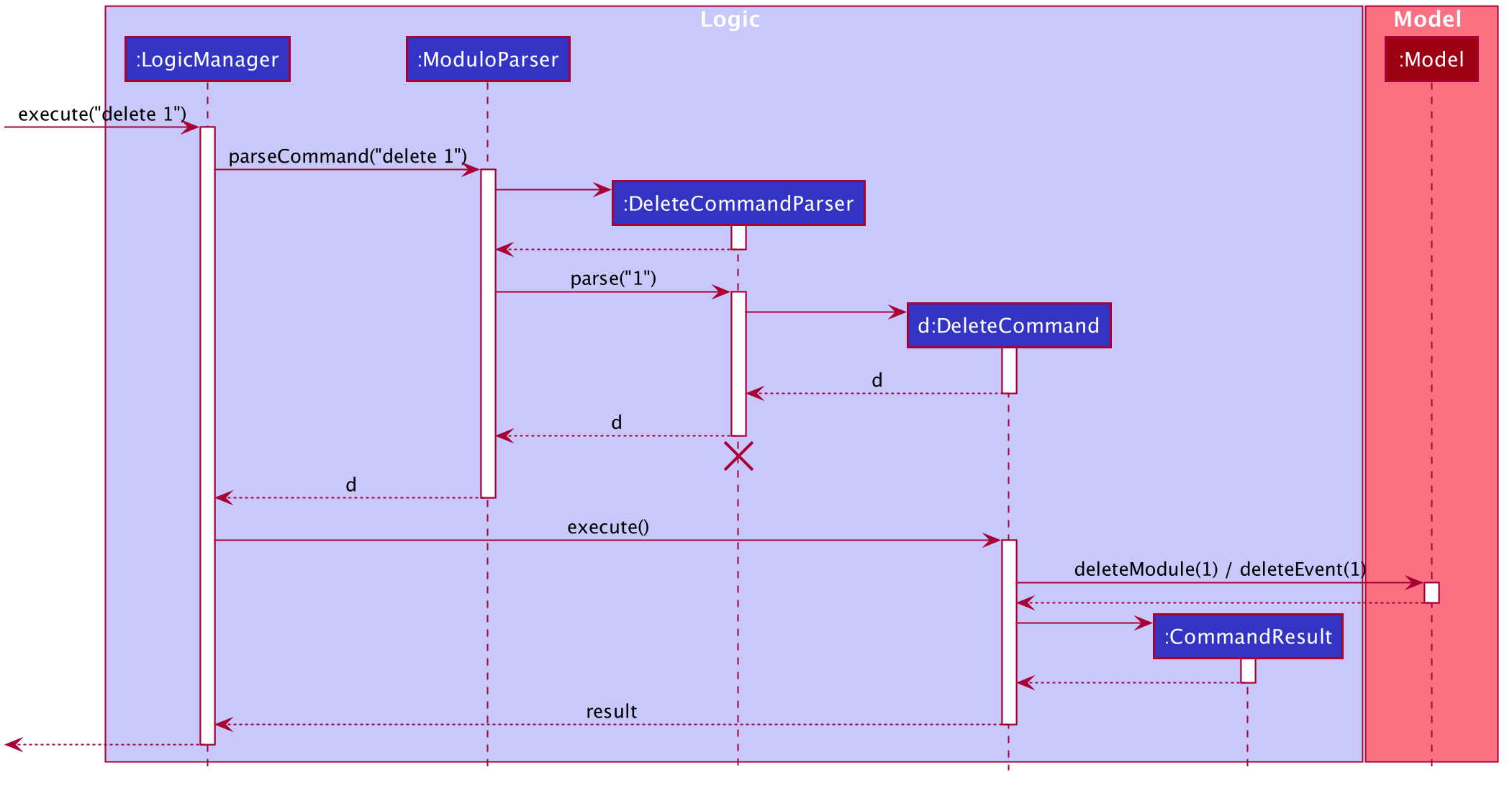
delete 1
Command
The lifeline for DeleteCommandParser should end at the destroy marker (X) but due to a limitation of PlantUML, the lifeline reaches the end of diagram.
|
Module / Event / Deadline Deletion Feature
This Deletion feature allows the user to delete modules, events or deadlines.
The user input is first retrieved by the MainWindow
class which is then passed to the LogicManager
via the execute
function.
LogicManager
will call the parseCommand
function of ModuloParser`, which will parse the input to create a temporary DeleteCommandParser
object, parsing the input and returning a DeleteCommand
.
The command will then be executed in the LogicManager
, returning a CommandResult
object which will then be returned as feedback to the user.
Given below is a sequence diagram to show how the delete
feature parsing an input of '1' behaves at each step:
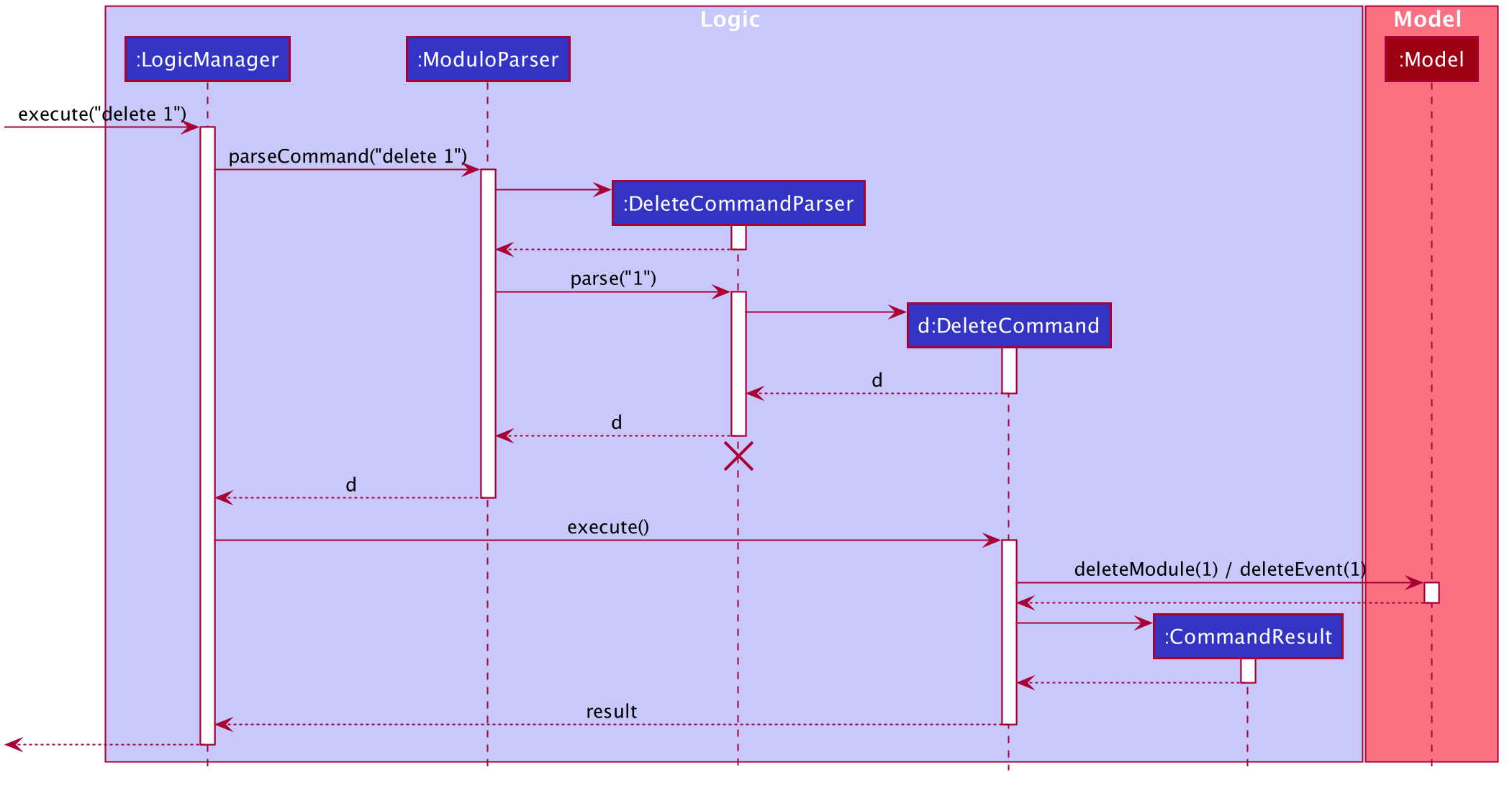
Given below is an activity diagram to show how the delete
operation works.
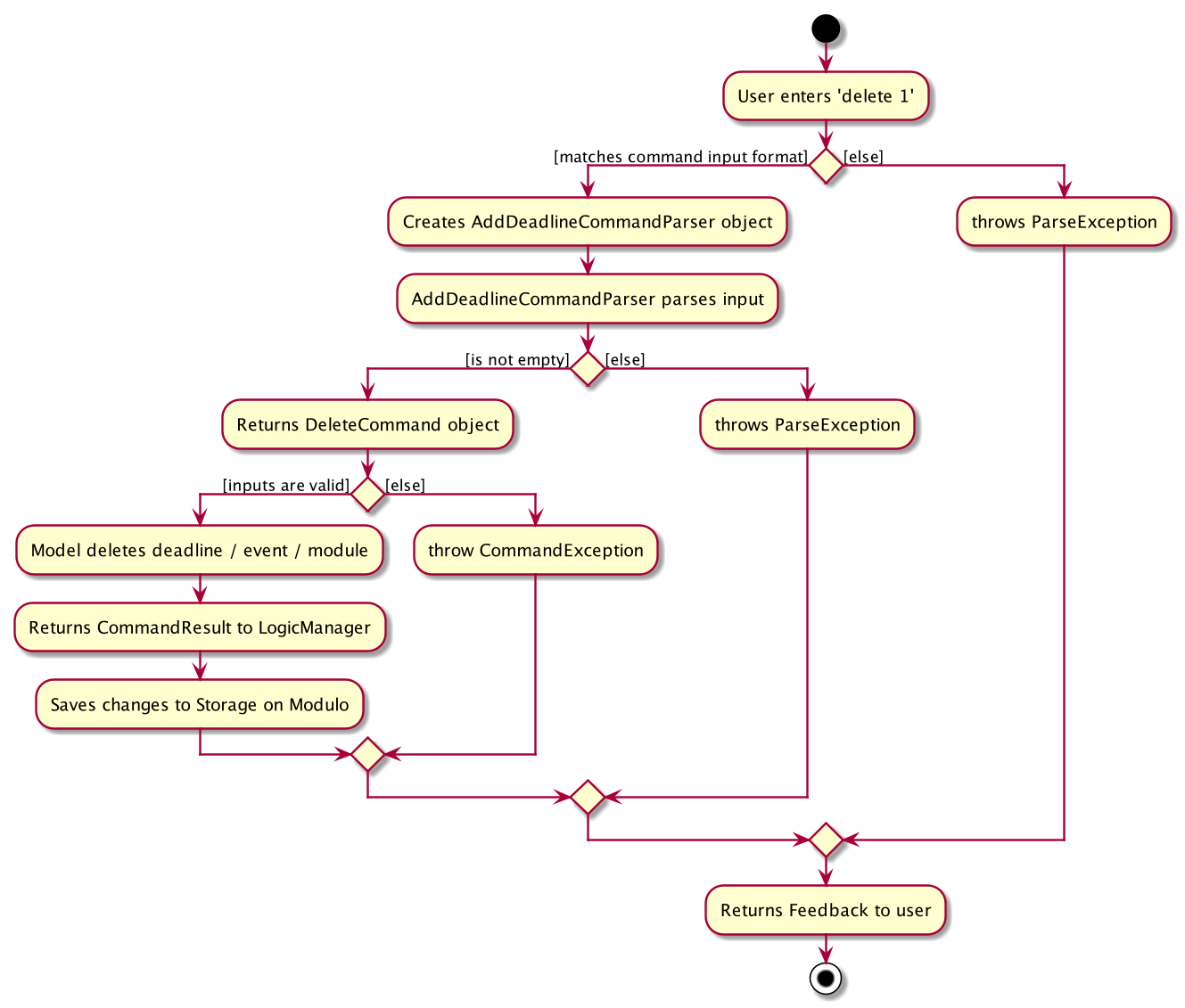
Design Considerations
Aspect: How delete
may be executed
-
Alternative 1 (current choice): User can delete multiple items using the command at the same time.
-
Pros: Allows the user more flexibility in deleting modules and events instead of just deleting them one by one. For example users can choose to delete one, many or even all modules and events, depending on their command input.
-
Cons: This has caused the code to become more complex in dealing with the many possible inputs and scenarios, leading to a higher amount of potential errors.
-
With respect to deadlines, users can only delete one or all deadlines of a particular event. This is to avoid any confusion and unnecessary complexity which may be caused by deleting deadlines across all events, especially since the user may only observe deadlines of one event at any one time and do not have access to the overall list of deadlines. |
-
Alternative 2 : User can
delete
only one or all items.-
Pros: Reduces the lack of potential errors due to the decreased complexity of the code.
-
Cons: It is less intuitive for the user as users are now limited by deleting one or all the events / modules / deadlines.
-
-
Future Extension: Modulo can have another UI panel to display every deadline that needs to be done a certain week. The user can then use the
delete
command to delete multiple deadlines at one go, making it more convenient for the user.
Use case: UC03 - Delete a module / event / deadline
-
User enters
delete
followed by an input ordelete d/
followed by an input for deadlines. -
Modulo parses the input.
-
The input is parsed and the relevant item is deleted.
-
Modulo displays the item or items that have been deleted. Use case ends.
Extensions
-
1a. User enters an invalid input.
-
1b. Step 2 takes place.
-
1b1. Modulo informs the user that the item the user wants to delete does not exist.
-
1b2. User enters a new item to deleteadd
Use case resumes from step 1.
-